ES6 Features | Parameter Handling
June 24, 2020 6:08 pm Comments Off on ES6 Features | Parameter HandlingES6 brought a lot of new and exciting features to JavaScript. These features are making the lives of developers easier than ever before by allowing them to code in a more elegant and concise way.
Among other features, ES6 also included multiple upgrades in parameter handling and that’s what we will be reviewing today.
In this article, we’ll be discussing the three most-awaited parameter handling features introduced in ES6:
- Default parameters handling
- Spread operator
- Rest parameter
Parameter and Arguments
There are two basic concepts you must be familiar with before diving deeper into parameter handling:
Parameters: the variable names declared at the time of the function definition.
Arguments: the actual values passed down to a function when it’s called.
Default Parameter Handling
In any well-coded program, the developer must account for all scenarios. How the function will work if the value is not passed, or if the value is invalid, etc.
In JavaScript, the default value for function parameters is ‘undefined’.
The default parameter handling feature enables the default values for named parameters if no value or ‘undefined’ is passed. This way, even if an invalid value or no value is passed, the function still works using the default parameter.
The use of modules in JavaScript prompted the need for default values. As default modules must be smart enough to handle a condition where a value is not provided.
Before ES6
Before default parameters in ES6, the default values were typically assigned using the OR (||) operator.
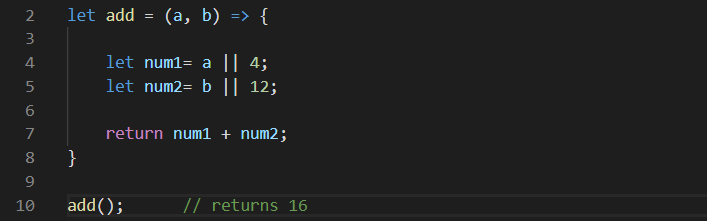
What’s Wrong with this approach?
The logic can fail if you explicitly needed the values to be false. It’s of course an edge case, but it shows that there is a gap in the logic.
Important: In the example above (and nearly all other examples in the article), we’ll be using the arrow functions. It’s another excellent ES6 feature. If you’re unfamiliar with the arrow functions, then it’s a good time to check our article on block scope and arrow functions.
After ES6
ES6 introduced default parameters in JavaScript. It enabled developers to assign a value to the parameters at the time of function definition. The default value will take over if no value or ‘undefined’ is passed.

In ES6, even if the value of the parameter was explicitly set to ‘undefined’, the function will still use the default value.
Each time a function is called, the default parameter is re-evaluated and a new object is created. This applies to functions and variables too.
The parameter values are assigned left-to-right. If you set one default parameter and pass only one value with the function call, then the value passed at the time of function call will overwrite the default value, and the second parameter will still be undefined.
Spread Operators
Spread operator denoted by three dots (…) takes an iterable such as an array or a string and as the name suggests, spreads its elements onto another array, object, or even as arguments of a function.
In a nutshell, the spread operator expands an array into its elements.
With the spread operator, we can perform the following operations:
- Concatenate (combine) arrays
- Copy one array to another
- Pass an arbitrary number of arguments to the function
It’s the exact opposite of the REST syntax.
In ES6, the Spread operator worked only with an array. Rest/Spread Properties for ECMAScript proposal added spread properties to the object literals in ES2018.
Before ES6
The spread operator offered a more concise way to:
- Combine or copy arrays
- Passing array as an argument
In Function Calls
The apply () function was used to pass array elements as arguments to a function.
In the following example, we’ll pass an array as a function argument using the apply method.

The apply() method gets the job done but it’s quite complicated.
In Array Literals
Before the spread operator, copying or combining arrays used to require a combination of array functions such as push(), concat(), and splice(). This would make the code messy and difficult to read.
There was also an issue of copying the ‘array elements’ vs. copying ‘reference to the arrays’. For example, if we copy one array ‘arr’ to another ‘arr2’ using the assignment operator, it will assign arr to arr2. Now, if we make changes in arr2, then arr will be mutated as well.

After ES6
Compared to the apply function, the spread operator offers a more precise way to pass array elements as arguments. See the example below:

The spread operator is (kind of) an all-in-one solution for copying, combining, or mutating arrays in different ways.
In the following example, we’ll copy an existing array to another using the spread operator.
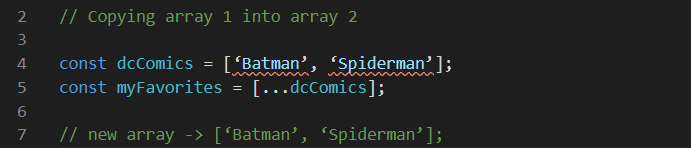
You can also concatenate two arrays easily using the spread operator.
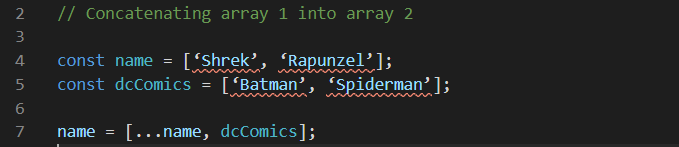
Rest Parameters
Many built-in JavaScript functions (such as Math.max) support an arbitrary number of arguments. The question here is:
How does it work?
How can we create a function that accepts an arbitrary number of arguments?
Rest parameters!
The Rest parameter enables us to represent an indefinite (or arbitrary) number of arguments as an array.
The syntax for the Rest parameter (…) is identical to the spread syntax but the concept is different or opposite (to be exact). A few important things about the Rest parameters are:
- In the case of more than one parameter, only the last parameter can be the Rest parameter.
- Rest parameters can only be used once in the function definition
- Array methods can be used on the Rest parameter, but not on the arguments object
Before ES6
An array-like object ‘argument’ was available to the function definition, in the previous versions of ECMAScript. It contained the values of arguments and could be accessed through the index (like an array).

The problem with the argument object is that array functions cannot be directly applied to the arguments. Also, argument objects are not available in the arrow functions.
After ES6
Rest parameters were introduced in ES6 to represent function arguments as an array. The arrow functions cannot use the argument object. Also, The rest operator is much easier to use as compared to the argument object.
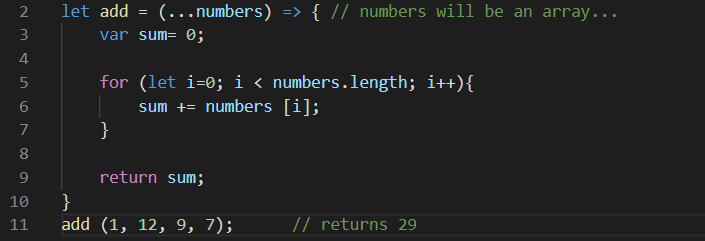
Array functions can be applied directly to the Rest parameters.
In this example, we’ll use the array of the same number from the previous example and apply the array function sort() on it. The function will return a sorted array of numbers.
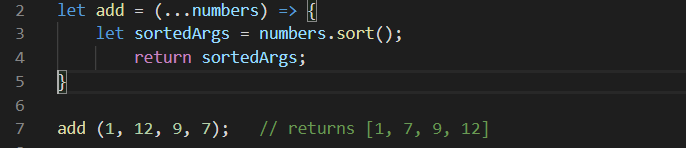
Do not confuse Rest parameters with REST (Representational State Transfer) web services. They’re two totally different concepts.
Reading through the article, you must’ve realized how ES6 solved many previous issues regarding parameter handling in JavaScript. Make sure to practice them in your code.
We’ll discuss more cool ES6 features in the upcoming article until then, don’t forget to try default parameters, and spread and rest syntax in your code!
Categorised in: Javascript
This post was written by Nearly Done