Modules and Promises in ES6
August 25, 2020 9:20 am Comments Off on Modules and Promises in ES6JavaScript was first introduced on December 4, 1995, as a scripting language. Considering it was just supposed to make web pages dynamic, it started pretty small. More features were added (and honed) along the way through the ECMAScript versions.
In this article, we will discuss two ES6 features called promises and modules. These two ES6 features have made programming complex tasks in Javascript more organised and easier.
Promises
Promises are a rather advanced topic in JavaScript. To understand promises, first, you need to be familiar with the concept of asynchronous programming in JavaScript.
Asynchronous JavaScript
JavaScript is a single-threaded programming language, which means that in a JavaScript program, only one thing can happen at a time. That’s not exactly convenient when we are working on complex logic.
To solve this issue, asynchronous JavaScript comes into play. Using asynchronous concepts such as promises, callbacks, and async/await, you can perform complex tasks that require long waits without blocking the flow of the program.
What is a Promise in JavaScript?
A promise in JavaScript is an object that represents the eventual completion (or failure) of an asynchronous operation with its (final) value.
The ES6 promise constructor takes a function which can take up to two parameters. Callback functions resolve() or reject() to indicate the success or failure of the promise.
You can declare a standard script as a module by including type=” module” in the <script> element in the HTML document (refer to the example below).

States of Promise
JavaScript promises are in one of the three following states:
- Pending: initial (first) stage
- Fulfilled: operation completed successfully
- Rejected: operation failed
Pending
Initially, a promise is in the stage of pending which means that it is waiting for something to resume its normal program flow. Now a promise is either fulfilled or rejected.
Fulfilled
A promise is fulfilled if it’s no longer pending. Which means that it has either been resolved or rejected. A promise is fulfilled by calling the resolve() method.
A promise can not be resettled once it has been settled. Calling resolve() or reject() again will have no effect. In a nutshell, a resolved promise is immutable.
Rejected
A promise is rejected if the operation fails. A promise is rejected by calling the reject() method.
How to Use Promises in ES6
To help you understand promises, let us consider a scenario where the program will wait for two seconds before sending a message to the user.
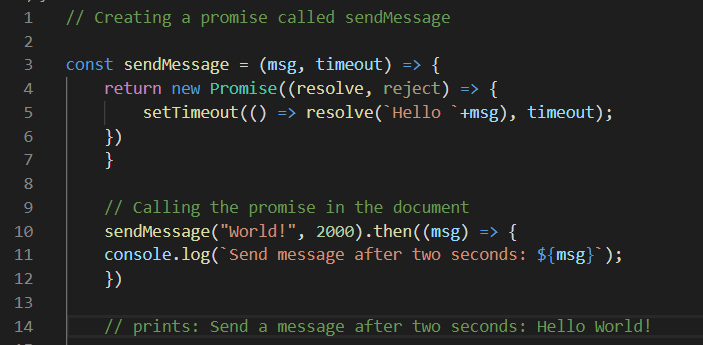
In the example above,
- The sendMessage call will wait for two seconds before sending the “Hello World” message.
- The setTimeout() method will create the delay and call the resolve() method afterward
- The function only takes the resolve() parameter only
- The resolve() parameter value is then passed through the callback function attached with .then().
Modules
As we discussed above, the JavaScript programs used to be shorter aimed at making web pages more interactive. In a complex JavaScript program, you can use modules to distribute the code into different parts. Modules organise code and make it easily accessible and readable.
A module in JavaScript is just a file. If a project contains three JavaScript (.js) files then each file is a distinct module. We can use import/export to exchange functionality between different modules.
Exporting Values
The import keyword labels variables and functions in one script that are accessible in another script.
In the following, we will export a variable and a function from modules/math.js script.

Importing Values
The export keyword allows one script to import functionalities from another script.
In the following example, we will import modules/math.js file into the main script.

Default Export Vs Named Export
In the example above, we have used named exports. Each item (function and variable) is referred to by its name upon export. The same name is used in the other script where it’s imported.
The default export is another kind of export aimed at making it easier to have a default function provided by a module in JavaScript.
To export a function as default, we use the keyword export default before the object name.
For Example:

We can also prepend export default in front of a function and make it an anonymous function.
For Example:

Notice the lack of curly braces in the examples above. It’s because there is only one default export allowed per module. In the above example, we know that the square is the default module.
Difference Between Standard Scripts and Modules
Modules are scripts but there are a few important differences between modules and scripts to keep in mind.
- Modules might behave differently as compared to the standard scripts. It’s because modules automatically execute in strict mode.
- Developers often face CORS errors during local testing due to JavaScript code security requirements. The alternate (and best course) is to use a server for testing.
- Modules are deferred automatically.
- Modules are executed only once in a script
- Module features are imported into the scope of a single script. You cannot access module features in the global scope.
Modules are a comparatively new but easy concept to understand. Whereas, promises are a quite complex concept. But both promises and modules have made coding in JavaScript more organised and clean. Make sure that you try both of these interesting ES6 features in your code to add extra and interesting functionality.
Tags: asynchronous javascript, ES6, es6 modules, es6 promises, ES6features, JavaScript, modules, promises
Categorised in: Javascript
This post was written by Nearly Done